Java Tic Tac Toe Program Code Using Arrays
A Beginner Tic-Tac-Toe Class for Java Posted on 04/27/14 Desktop Programming, General Discussion, Programming Theory Around this time each year we mentors on Dream.In.Code see a bunch of students and beginners, attempting to do there final programming projects, come on the boards asking for help with coding problems.
Still cannot figure out what im doing wrong. I have no idea how to get the CPU choice into the board. I did get the board to re display the updated board with the row and column the player selected. I need to have 4 methods and id like them to do exactly only this: 1) displayBoard (takes single passed parameter of 2d array representing current tic tac toe board. 2.) makeAMove (takes 2 passed parameters: Two dimensional array representing tictactoe board and player character value ('X' or 'O'). Updates array with valid row and column selected by player character. This method does NOT return anything, it simply updates the board array. 3.) hasWon (Takes 2 passed parameters, 2 dimensional array representing TicTacToe board and player character ('X' or 'O'). Return TRUE if player character has won, FALSE otherwise. 4.) boardFull (Takes single passed parameter of two dimensional array representing TicTacToe board and return TRUE if all cells are occupied, false otherewise. These area ll the methods, some of which i have done in an ineffiecient way (i know) but im trying to teach myself just the logic of it first without using any classes. Anyone that can either comment here it would greatly help as i feel stuck at this point.
} // end class
4 Answers
You just have to do this:
Serj tankian honking antelope mp3 download. Of course you would have to check beforehand that cell is not already occupied. If yes, then ask again for user input. I guess that wouldn't be that tough.
Apart from that, I would suggest you some improvements to your code:
Rather than having two players as
char
types, use anenum Player
, with two constants -X
andO
. And use aPlayer[]
instead.No need to initialize your array with
'1', '2', ..
. Now that they will benull
by default.Rather than having
board
as local variable, and passing it in all the methods, make it a field in your class.Currently your code is making just one move. Why? Also, you're not even using the return value of
hasWon()
andboardFull()
method.You can divide the
hasWon
method into 3 methods -hasWonHorizontal()
,hasWonVertical()
,hasWonDiagonal()
. This will avoid that longif
condition in the same method. And then call these 3 methods in sequence fromhasWon()
method.
Seems all you need to do is check to see if player/cpu has already taken that space. If not, you should just assign 'X' or 'O' to that element in the array.
You will probably want to have some kind of loop within your main() function to continuously allow players to move as well. The current implementation appears to only allow a player to make one move.
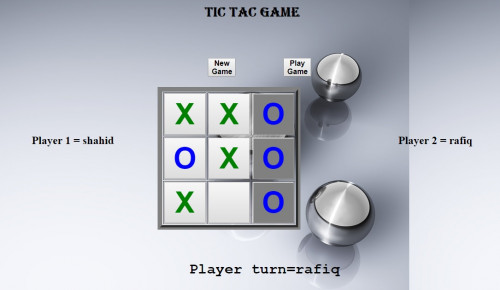
First a direct answer to your question:
To show the new board you can just call your displayBoard()
method again.
You should be careful with your scanner as it doesn't validate the input. If you get a number not in [0.2] you will get an ArrayIndexOutOfBounds here, so you might want to do something like
and the same for the column to prevent this. Also you can add checks for strings and so on.
Some other aspects for the game:
- Do you want to ask to continue before every move? If not, better move it to a new method and ask it once in the beginning.
- Make board, player and cpu class variables. (or see below)
- Use a loop around your game state, you already have a very nice 'state'-machine by providing your functions:
.
The best way to improve your game would be to make a TicTacToe class and a Main class. The TicTacToe class would hold the board, the player, the cpu and some methods to display the board and modify it, and maybe also to switch the player and so on.
The Main class would then just create the TicTacToe instance and run a main loop (the while-loop I posted above) which modifies it accordingly. But for now just go with your approach and maybe later you might want to improve it further. Then you can come back and think about it.
Not the answer you're looking for? Browse other questions tagged java or ask your own question.
I am currently writing a basic Java program for a tic tac toe board. I am trying to build this without arrays, but almost every online help uses them. I have much of the code written, but I am hitting a wall on creating a method to print the updated board with the characters (X or O) in the board. I am also supposed to create a game that plays against the computer, but does not have to be anything other than random. I know I can use Math.random to select the computer move, but I am not certain how to construct using the code I have. The missing pieces are:
- After a valid input is received the symbol is placed and the board is printed to the console-computer randomly placing its ‘O’ on a non-occupied field and include checking for a winner-create a game loop that continues until there is a winner
import java.util.Scanner;
//Heather Richards author
public class Assignment3TicTacToe {
} }
Thanks for your help!!!!!!!!!!!!!!!